HTML Basics
What You Need To Know
- How to set up a basic HTML page and what each element does
- How to view your page in a web browser
- How to create basic text, list, link, and image elements
Resources
Getting Set Up
Create an HTML (HyperText Markup Language) file on your computer (specifics discussed in class), naming it index.html. In addition to having this file open in your text editor, you can open it in your browser (Chrome, Firefox, or Safari) and refresh periodically to preview the changes you make.
Then, copy the following code into your file to setup the basic page structure.
<!DOCTYPE html>
<html>
<head>
<title></title>
<meta charset="utf-8">
<meta name="viewport" content="initial-scale=1.0, width=device-width">
<link rel="stylesheet" href="style.css">
</head>
<body>
</body>
</html>
Understanding the Code
An HTML page is made up of elements, with most elements having an opening and closing tag. Elements are often nested within other elements. For instance, the <head>
and <body>
elements are nested within the <html>
element.
The <html>
element contains the entire page – both the metadata about the page and the actual page content. <head>
contains metadata such as the title of the page, the language it uses, and links to dependencies. <body>
contains the actual code that is visible in the browser.
The DOCTYPE
and character encoding (charset
) will be the same on all our pages. The DOCTYPE
tells your browser to interpret the page as HTML, and the character encoding tells your computer what set of characters should be used on the page (more information here).
Finally, the <title>
element lets you set the text that will show up in the browser menu bar or tab when it opens your page. Make sure to choose something short and descriptive - don't use the same thing on every page of your site.
(The <meta>
elements mentioning viewport
and stylesheet
will be covered later as we begin to work with them.)
Basic Elements for Content
The following elements will get you started adding content to your page. To start with we will just see how your browser styles these elements by default.
Text
The <p>
element is used for paragraphs of text. Each paragraph should have its own opening and closing tags.
<h1>
through <h6>
represent different levels of headings. It's best to think hierarchically when thinking about which heading to use – the main heading on your page should be <h1>
, the subheadings <h2>
, the headings under each subheading <h3>
, etc. These are not meant to be used in numerical order.
<strong>
creates bold text and <em>
creates italicized text. These are best used within paragraphs, list items, etc.
Finally, <hr>
creates a horizontal line, useful for creating separation between sections of text. Note that this element doesn't have a closing tag.
Here is some example code using these elements:
<h1>A main heading</h1>
<h2>A subheading</h2>
<p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. <strong>Curabitur porttitor bibendum risus,</strong> ac consectetur lectus tristique sed. Nunc fringilla elementum lorem molestie ullamcorper. Integer eu justo dolor.</p>
<hr>
<h2>Another subheading</h2>
<p>Morbi enim diam, sodales quis egestas ac, aliquet nec ligula. Quisque tempus tristique imperdiet. Etiam sodales leo vitae lacus congue at bibendum orci consequat. <em>In nec odio odio, quis iaculis purus.</em></p>
And how this code looks in the browser:
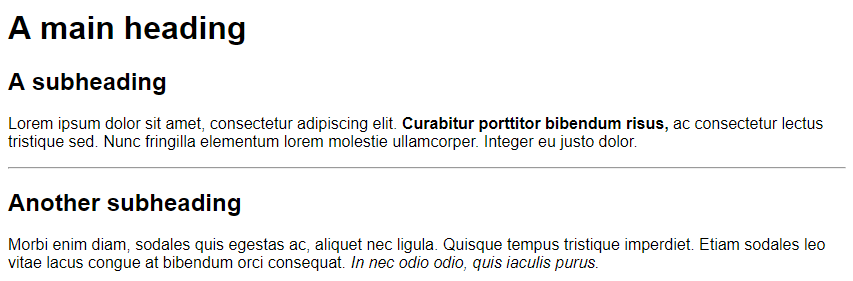
Lists
Lists use two elements – one to denote what type of list it is, and another element for each individual list item. An unordered (bulleted) list would look like the following:
<ul>
<li>List item 1</li>
<li>List item 2</li>
<li>List item 3</li>
</ul>
Ordered (numbered) list use the same basic structure except for an <ol>
instead of <ul>
.
Links
Links, or hyperlinks, to other pages and resources on the internet are created using the <a>
(anchor) element. In order to determine where the link will lead to, you will need to use the href
attribute.
<a href="http://example.com">An example link</a>
Pay close attention that you format your attribute appropriately, and don't forget to include http://
at the beginning of your links. (Links to email addresses use mailto:
instead, although it's generally not a good idea to directly link to email addresses unless you'd like to get more spam.)
Images
The <img>
element uses an attribute to locate an image file and embed it on the page. You should also define an alt
attribute that describes the image (for use in screen readers, etc.).
<img src="test.jpg" alt="A sample image as an example">
In Closing
These few elements will go a long way in helping you create the content of your websites. Next up, we'll cover how to change the appearance of these elements and create a design for your site.